golang 으로 조건문을 사용할 때 주의할점이 있다.
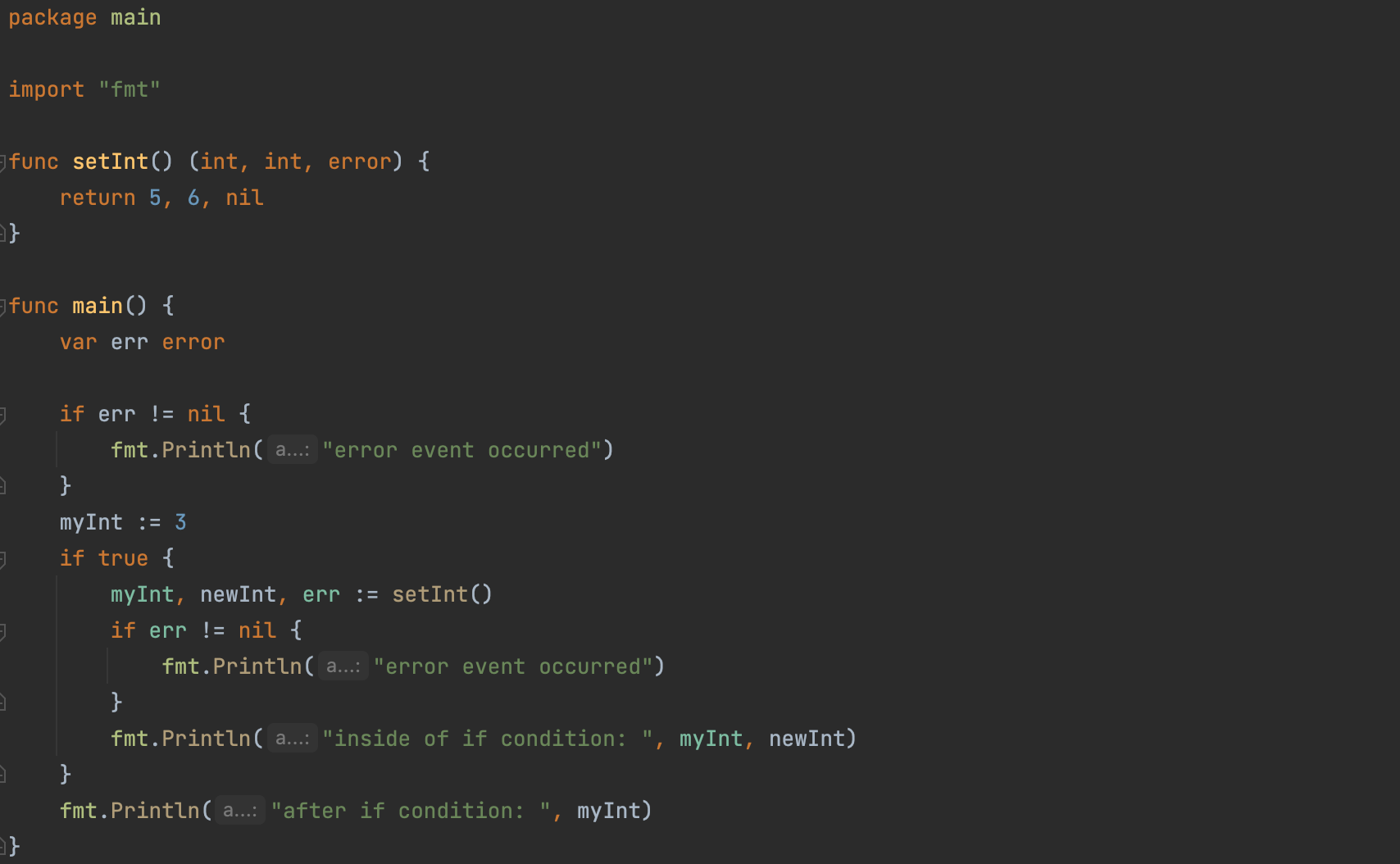
package main
import "fmt"
func setInt() (int, int, error) {
return 5, 6, nil
}
func main() {
var err error
if err != nil {
fmt.Println("error event occurred")
}
myInt := 3
if true {
myInt, newInt, err := setInt()
if err != nil {
fmt.Println("error event occurred")
}
fmt.Println("inside of if condition: ", myInt, newInt)
}
fmt.Println("after if condition: ", myInt)
}
위와같이 변수(myInt
)를 if 조건문 밖에 선언해서 초기값을 갖도록 하고 특정 함수를 통해 해당 값에 변경이 있었을 때, :=
operator 를 이용하여 선언과 동시에 값할당을 하게되면 해당 변경사항은 if condition 안에서만 적용된다.
그렇다고 조건문에서 :=
를 =
로 변경하면 newInt
가 선언되지 않은 변수기때문에 undefined error 가 발생하게된다.
이러한 현상을 해결하기위해서는 myInt, newInt 를 모두 if condition 밖에서 선언하고 조건문 내에서 =
operator 를 사용하도록 아래와 같이 변경한다.
...
myInt, newInt := 3, 5
if true {
myInt, newInt, err = setInt()
if err != nil {
fmt.Println("error event occurred")
}
...
반응형
'golang' 카테고리의 다른 글
golang gin API + swagger 적용하기 (0) | 2022.11.15 |
---|---|
Go gin middleware 적용하기 (0) | 2022.11.14 |
API server를 위한 go Gin 설치하고 사용하기 (예제) (0) | 2022.04.14 |
[golang] go routine과 chan 이용한 메세지 입력 (0) | 2022.03.15 |
[golang] defer, panic, recover (0) | 2022.02.28 |